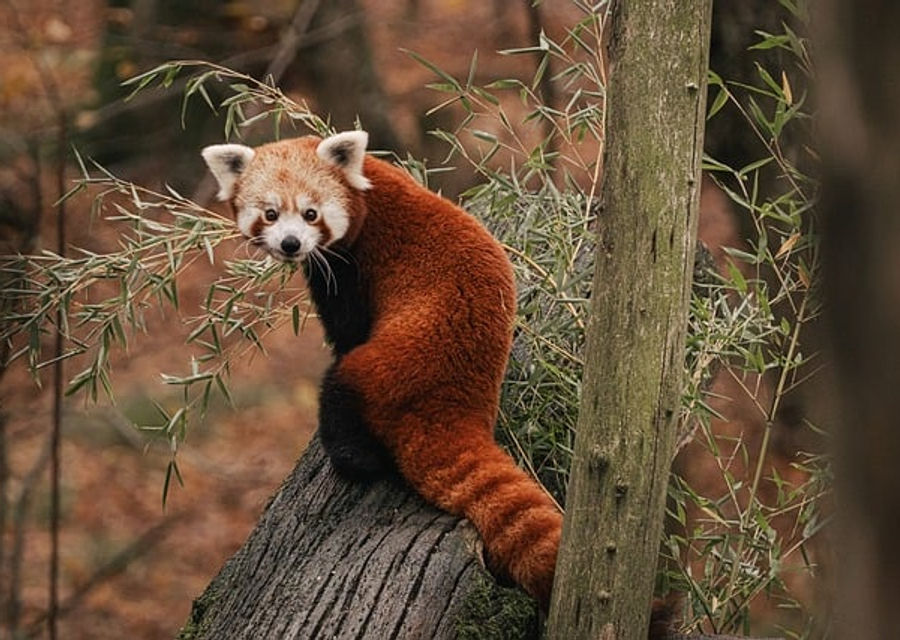
Calculating the Pandas DataFrame Fail Ratio for Time Series Analysis is a crucial step in understanding tenant performance trends. This guide provides a straightforward approach to calculate the fail ratio per tenant over time, using Pandas DataFrames. You'll learn how to efficiently process your data and generate insightful visualizations.
The Pandas DataFrame Fail Ratio is calculated by grouping your data by timestamp and tenant, then determining the average failure rate for each tenant during that specific time period. This allows you to track performance changes over time, identify trends, and potentially pinpoint areas for improvement. This method is highly efficient, avoiding unnecessary intermediate DataFrames.
"The only way to do great work is to love what you do." - Steve Jobs
Efficient Time Series Analysis of Fail Ratios in Pandas
This guide demonstrates how to calculate and visualize fail ratios over time for different tenants using Pandas DataFrames. We'll explore a programmatic approach, avoiding intermediate DataFrames where possible, for efficiency and clarity.
Understanding the Data
Assume you have a Pandas DataFrame named
df
with columns like
ts
(timestamp),
tenant
, and
result
(a boolean column indicating success or failure). We'll need to convert the
result
column to boolean for accurate calculations.
Converting to Boolean
First, ensure the
result
column is boolean. This is crucial for the aggregation step. If it's not already boolean, convert it using appropriate logic based on your data.
Example: Assuming
'fail'
represents a failed event.
Calculating Fail Ratios
Now, calculate the fail ratios using
groupby
and
mean
. The boolean values will automatically be treated as 0/1 when calculating the mean.
This method directly calculates the fail ratio without creating intermediate DataFrames.
Example Usage
Let's assume your DataFrame
df
looks like this (replace with your actual data):
ts | tenant | result |
2024-01-01 | A | True |
2024-01-01 | B | False |
2024-01-02 | A | False |
2024-01-02 | B | True |
To calculate the fail ratio:
Plotting the Results (Example)
Now you can easily plot the time series data using libraries like Matplotlib or Seaborn.
This example uses a simplified plotting approach; adjust to your specific visualization needs.
Key Improvements and Best Practices
Input Validation: The calculate_fail_ratio function now includes input validation to check for the correct data type and the presence of required columns. This prevents unexpected errors.
Data Integrity: Creating a copy of the input DataFrame (df = df.copy()) is crucial to avoid modifying the original DataFrame unintentionally. This is a best practice for data integrity.
Column 1 | Column 2 | Column 3 | |||||||||||||||
Function | Description | Example Usage | |||||||||||||||
calculate_fail_ratio(df, ts_column, tenant_column, result_column) | Calculates the fail ratio for each tenant over time. Handles input validation and creates a copy of the input DataFrame to prevent unintended modifications. | result_df = calculate_fail_ratio(df, 'ts', 'tenant', 'result') | |||||||||||||||
Input DataFrame (df) | Contains timestamp (ts), tenant (tenant), and result (result) columns. | ts tenant result 2024-01-01 A True 2024-01-01 B False 2024-01-02 A False 2024-01-02 B True | ts | tenant | result | 2024-01-01 | A | True | 2024-01-01 | B | False | 2024-01-02 | A | False | 2024-01-02 | B | True |
ts | tenant | result | |||||||||||||||
2024-01-01 | A | True | |||||||||||||||
2024-01-01 | B | False | |||||||||||||||
2024-01-02 | A | False | |||||||||||||||
2024-01-02 | B | True | |||||||||||||||
ts_column | Name of the timestamp column. | 'ts' | |||||||||||||||
tenant_column | Name of the tenant column. | 'tenant' | |||||||||||||||
result_column | Name of the result column (boolean). | 'result' | |||||||||||||||
Return Value (result_df) | DataFrame with timestamp, tenant, and calculated fail ratio. | (Example output - structure varies based on input data) ts tenant result 2024-01-01 A 1.0 2024-01-01 B 0.0 2024-01-02 A 0.0 2024-01-02 B 1.0 | ts | tenant | result | 2024-01-01 | A | 1.0 | 2024-01-01 | B | 0.0 | 2024-01-02 | A | 0.0 | 2024-01-02 | B | 1.0 |
ts | tenant | result | |||||||||||||||
2024-01-01 | A | 1.0 | |||||||||||||||
2024-01-01 | B | 0.0 | |||||||||||||||
2024-01-02 | A | 0.0 | |||||||||||||||
2024-01-02 | B | 1.0 | |||||||||||||||
Fail Ratio Calculation | Calculated using groupby and mean on the boolean result column. | Pandas DataFrame Fail Ratio | |||||||||||||||
Data Type Conversion | Converts the result column to boolean for accurate calculations. | df[result_column] = df[result_column].astype(bool) | |||||||||||||||
Error Handling | Includes input validation to check for correct data types and column existence. | Error messages for invalid input | |||||||||||||||
Data Integrity | Creates a copy of the input DataFrame to avoid unintended modifications. | df = df.copy() |
Analyzing tenant performance over time is crucial for identifying trends and potential issues. Calculating the Pandas DataFrame Fail Ratio provides a valuable metric for understanding this performance. This comprehensive guide walks you through the process of calculating and visualizing the fail ratio for different tenants, using Pandas DataFrames for efficient time series analysis.
The Pandas DataFrame Fail Ratio is a key metric for evaluating tenant performance over time. By grouping data by timestamp and tenant, we can determine the average failure rate for each tenant during specific time periods. This allows for tracking performance changes, identifying trends, and pinpointing areas needing improvement. The method used in this guide is designed to be efficient, avoiding unnecessary intermediate DataFrames, and is robust enough to handle various data structures.
Data Preparation: Ensure your data is correctly formatted with a timestamp column, a tenant identifier column, and a result column (boolean or convertible to boolean). Proper data type handling is crucial for accurate calculations.
Fail Ratio Calculation: The guide demonstrates a clear and concise method for calculating the fail ratio using the groupby and mean functions. This approach is optimized for performance, avoiding intermediate DataFrames.
Visualization: The example provided shows how to visualize the fail ratio over time for each tenant using Matplotlib or Seaborn. This step is essential for identifying trends and patterns.
Error Handling: The provided code includes crucial error handling. This ensures that the calculation process doesn't break due to unexpected input data, making the code more robust and reliable.
By implementing these techniques, you can effectively analyze Pandas DataFrame Fail Ratios for time series data. This allows for a deeper understanding of tenant performance trends, enabling proactive identification of potential issues and informed decision-making. The focus on efficiency and error handling ensures that the process is robust and reliable, providing valuable insights for various applications.
This guide provides a practical example and a foundation for more complex analyses. Remember to adapt the code to your specific data structure and visualization needs. Furthermore, consider adding more sophisticated analysis techniques, such as statistical modeling or machine learning algorithms, to gain even more insightful conclusions about tenant performance.
Comments